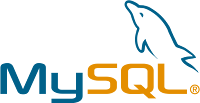
functions. MySQL is the default database which is created while initial installation of the database. Using the following steps you will be able to create a user for a specific database and also if you want you can assign a password to it.
1. First of all create a user in the MySQL Database after log into it, using the following query:
mysql> CREATE USER 'abcd'@'localhost' IDENTIFIED BY '1qazXSW@';2. Grant all or specific privileges to the new user and also assign a password as required.
mysql> GRANT ALL PRIVILEGES ON abcd_designs.* TO 'abcd'@'localhost';or
mysql> GRANT SELECT, INSERT, UPDATE, DELETE, CREATE, DROP, ALTER, INDEX ON abcd-designs.* TO 'abcd'@'localhost' IDENTIFIED BY 'abcd@123';3. After performing all the above operations, in-order to tell the server to reload the grant tables, perform a flush-privileges operation. This can be done by issuing a FLUSH PRIVILEGES statement or by executing a mysqladmin flush-privileges or mysqladmin reload command.
A grant table reload affects privileges for each existing client connection as follows:
- Table and column privilege changes take effect with the client's next request.
- Database privilege changes take effect the next time the client executes a USE db_name statement.
Client applications may cache the database name; thus, this effect may not be visible to them without actually changing to a different database or flushing the privileges.
- Global privileges and passwords are unaffected for a connected client. These changes take effect only for subsequent connections.
mysql> FLUSH PRIVILEGES;If there is a "-" hyphen between the names then we can use backtick to bypass it like `abcd-designs`
Attributes:
Database name = abcd_designsUser name = abcd
Password = abcd@123